10 common mistakes that Django developers make
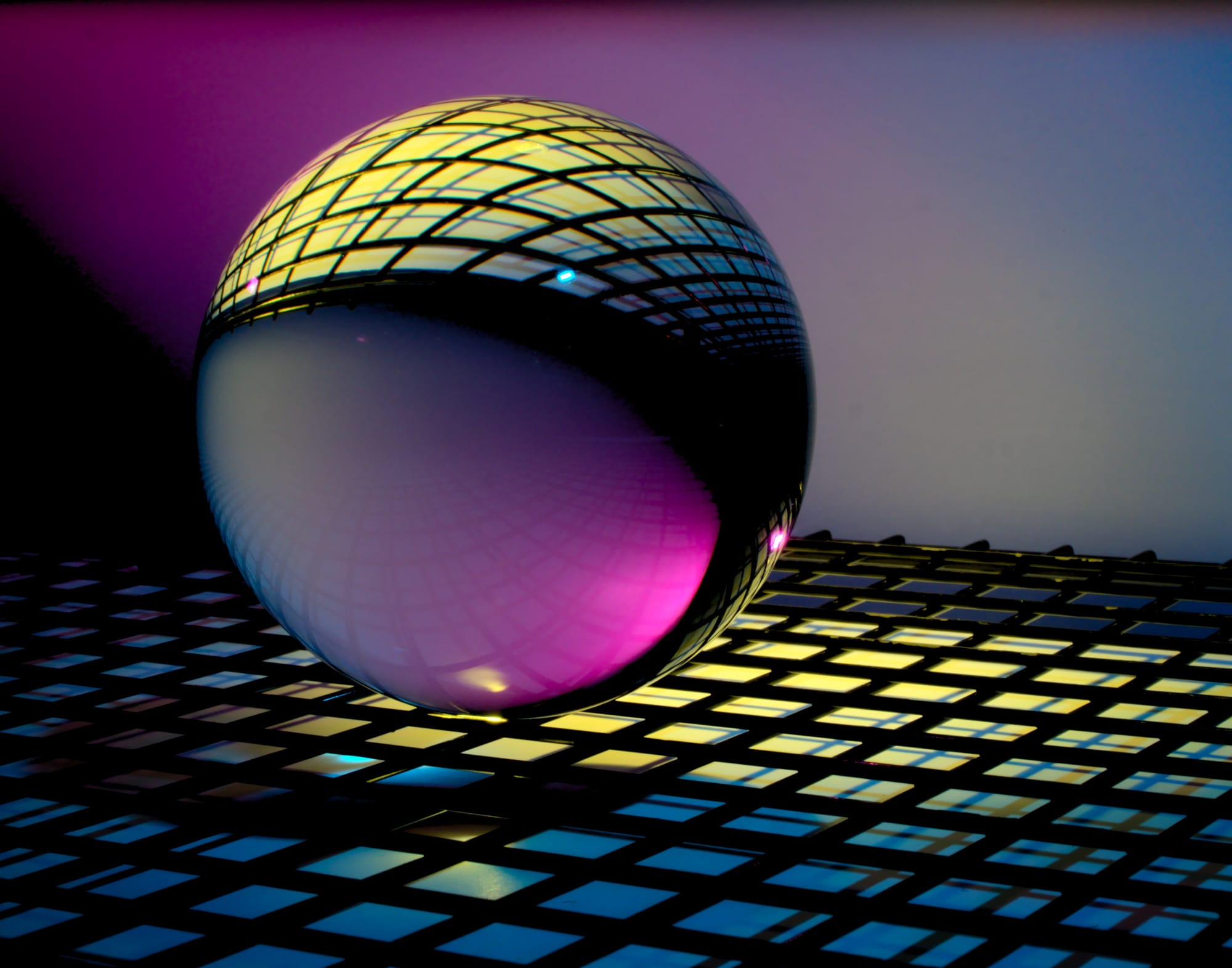
Django is an open-source web development framework, grounded in the world's most used language Python, that's designed to make building web applications fun, simpler and more structured.
Its main attractions lie in its powerful features, like an intuitive and automated admin interface, Object Relational Mapping (ORM) for database interactions, efficient routing, and flexible templating options. These tools collectively make Django an ideal choice for crafting well-organized and adaptable web apps.
Django also comes with an extensive community and ecosystem of apps and people that constantly work to increase the collective efforts in building the best framework for web development.
Benefits of Django:
- Supports major databases.
- Simplifies SQL operations with the ORM
- Popular for its convenience and efficiency
- Has an amazing community
- Has a large ecosystem of apps and solutions
- Is widely used by startups and large organizations
Code Examples for Common Django Development Mistakes
-
Monolithic Structure
- Bad Example: A single Django app handling multiple functionalities like user management, payment processing, and content management.
# In a single app 'myapp' class User(models.Model): # User-related fields class Payment(models.Model): # Payment-related fields class Content(models.Model): # Content-related fields
- Good Example: Separate apps for each functionality.
# In 'users' app class User(models.Model): # User-related fields # In 'payments' app class Payment(models.Model): # Payment-related fields # In 'content' app class Content(models.Model): # Content-related fields
- Bad Example: A single Django app handling multiple functionalities like user management, payment processing, and content management.
-
Ignoring
requirements.txt
- Bad Example: Installing packages without tracking versions.
pip install django pip install requests
- Good Example: Using a
requirements.txt
file with specific versions.# requirements.txt django==3.2.5 requests==2.25.1
- Bad Example: Installing packages without tracking versions.
-
Outdated Python Functions
- Bad Example: Using function-based views.
def my_view(request): # Processing logic return render(request, 'template.html', context)
- Good Example: Using Class-Based Views.
class MyView(View): def get(self, request): # Processing logic return render(request, 'template.html', context)
- Bad Example: Using function-based views.
-
Imbalanced Code Structure
- Bad Example: Fat views, thin models.
# views.py def my_view(request): # Extensive business logic return render(request, 'template.html', context) # models.py class MyModel(models.Model): # Minimal logic
- Good Example: Fat models, thin views.
# models.py class MyModel(models.Model): # Extensive business logic # views.py def my_view(request): # Minimal logic, mainly handling request and response return render(request, 'template.html', context)
- Bad Example: Fat views, thin models.
-
Models Not Adding Indexes
- Bad Example: No indexes on frequently queried fields.
class User(models.Model): username = models.CharField(max_length=100) email = models.EmailField()
- Good Example: Adding indexes to important fields.
class User(models.Model): username = models.CharField(max_length=100, db_index=True) email = models.EmailField(db_index=True)
- Bad Example: No indexes on frequently queried fields.
-
Inconsistent Data Validation
- Bad Example: Mixed validation logic.
class UserForm(forms.ModelForm): # Form-specific validation class User(models.Model): # Model-specific validation
- Good Example: Consistent validation across models and forms.
class User(models.Model): # Centralized validation logic class UserForm(forms.ModelForm): class Meta: model = User # Reuse model's validation logic
- Bad Example: Mixed validation logic.
-
Reinventing The Wheel
- Bad Example: Writing custom code for features Django provides.
# Custom user authentication system def custom_authenticate(user): # Custom authentication logic
- Good Example: Utilizing Django's built-in features.
from django.contrib.auth import authenticate user = authenticate(username='john', password='secret')
- Bad Example: Writing custom code for features Django provides.
-
Overlooking Django's Management Commands
- Bad Example: Writing standalone Python scripts for database cleanups.
# standalone_script.py # Custom script for database cleanup
- Good Example: Using Django's management command structure.
from django.core.management.base import BaseCommand class Command(BaseCommand): def handle(self, *args, **options): # Database cleanup logic
- Bad Example: Writing standalone Python scripts for database cleanups.
-
Confusing STATICFILES_DIRS with STATIC_ROOT
- Bad Example: Misusing STATICFILES_DIRS and STATIC_ROOT.
STATICFILES_DIRS = [os.path.join(BASE_DIR, 'static')] STATIC_ROOT = os.path.join(BASE_DIR, 'static')
- Good Example: Correct usage.
STATICFILES_DIRS = [os.path.join(BASE_DIR, 'assets')] STATIC_ROOT = os.path.join(BASE_DIR, 'static')
- Bad Example: Misusing STATICFILES_DIRS and STATIC_ROOT.
-
Avoid using too many defaults in settings.py
APPEND_SLASH = True
Sometimes examples like above are necessary but often they just make the settings.py file more and more bloated. Try to think about how you can keep it lightweight.
These examples illustrate the importance of adhering to best practices in Django development to ensure efficient and maintainable code. More than anything try to think of keeping your codebase tidy at all times so that your colleagues and peers are not fatigued when they try to understand what code is doing.
Key Takeaways
- Utilize Django's features and best practices for effective web development.
- Avoid common pitfalls for increased application efficiency.
- Avoid complexity and keep things where they should be. Django comes with a very good structure and in most projects you just need to adhere to Django's best practices.
- Continuous learning and adapting to Django's capabilities are crucial for successful development. Try to understand where you need to add your own code versus use an existing solution that already does what you need it to do.